【PyTorch】代表的な活性化関数の使い方と一覧について

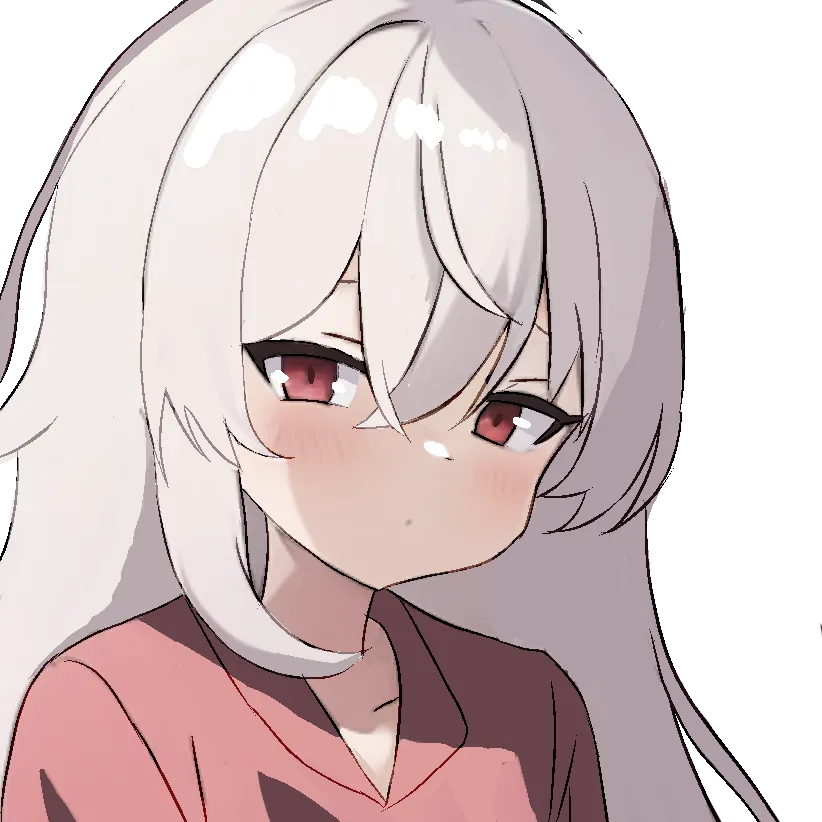

1. 活性化関数とは?
活性化関数(Activation Function)は、ニューラルネットワークの各ニューロンが受け取った入力をどのように変換して次の層に送るかを決定するルールです。ニューラルネットワークが非線形なデータを学習し、複雑なパターンや関係性を捉えるために必要不可欠な要素です。
PyTorchでは、ニューラルネットワークを構築する際にさまざまな活性化関数が用意されています。以下に、PyTorchでよく使用される代表的な活性化関数をいくつか紹介します。
2. ReLU (Rectified Linear Unit)
torch.nn.ReLU
ReLUは負の値をゼロに変換し、正の値はそのまま出力するシンプルな関数です。
数式: \( \text{ReLU}(x) = \max(0, x) \)
import torch
f=torch.nn.ReLU()
out=f(torch.randn(4))
print(out)
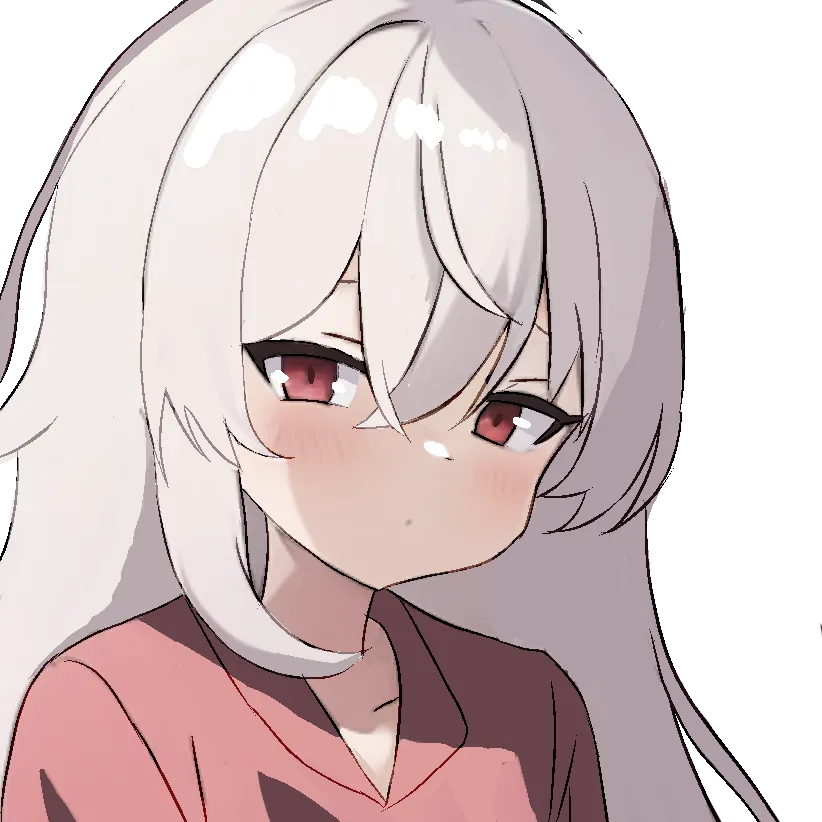

2.1. Leaky ReLU
torch.nn.LeakyReLU
Leaky ReLUは ReLUの改良版で、負の入力に対しても小さな傾きで値を出力します。
数式: \( \text{LeakyReLU}(x) = \begin{cases} x & x \geq 0 \\ \alpha x & x < 0 \end{cases} \) (\( \alpha \)は小さな正の値)
- 特徴:
- 負の入力にも情報を保持できる。
- Dying ReLU問題を軽減。
import torch
f=torch.nn.LeakyReLU()
out=f(torch.randn(4))
print(out)

2.2. ELU (Exponential Linear Unit)
torch.nn.ELU
ReLUの改良版で、負の値に対して指数的な減少を導入。
\( \text{ELU}(x) = \begin{cases} x & x \geq 0 \\ \alpha (e^x – 1) & x < 0 \end{cases} \) (\( \alpha \)はパラメータ)
import torch
f=torch.nn.ELU()
out=f(torch.randn(4))
print(out)
2.3. Softplus
torch.nn.Softplus
SoftplusはReLUと似た外形で滑らかにしたReLUのような関数。
数式: \( \text{Softplus}(x) = \ln(1 + e^x) \)
import torch
f=torch.nn.Softplus()
out=f(torch.randn(4))
print(out)
3. Sigmoid
torch.nn.Sigmoid
Sigmoid関数は入力値を0から1の範囲にマッピングする滑らかな関数です。
数式: \( \text{Sigmoid}(x) = \frac{1}{1 + e^{-x}} \)
import torch
f=torch.nn.Sigmoid()
out=f(torch.randn(4))
print(out)
4. Swish
torch.nn.SiLU
(Sigmoid-Weighted Linear Unit)
入力値をシグモイドで重み付けして出力する関数。
数式: \( \text{Swish}(x) = x \cdot \text{Sigmoid}(x) \)
import torch
f=torch.nn.SiLU()
out=f(torch.randn(4))
print(out)
5. Tanh (Hyperbolic Tangent)
torch.nn.Tanh
入力値を-1から1の範囲にマッピングする関数。
数式:\( \text{Tanh}(x) = \frac{e^x – e^{-x}}{e^x + e^{-x}} \)
import torch
f=torch.nn.Tanh()
out=f(torch.randn(4))
print(out)
6. Softmax
torch.nn.Softmax
ソフトマックス関数は入力値を確率分布に変換する関数で、多クラス分類タスクで使用されます。
数式: \( \text{Softmax}(x_i) = \frac{e^{x_i}}{\sum_{j} e^{x_j}} \)
出力の合計が1になるため、確率を表現可能。
import torch
f=torch.nn.Softmax()
out=f(torch.randn(4))
print(out)
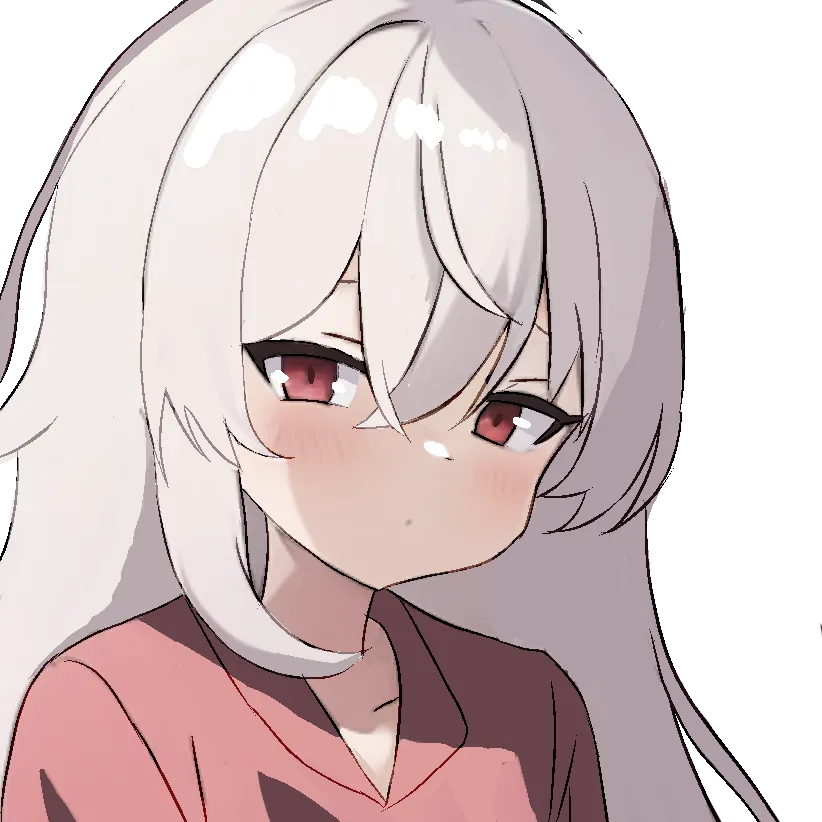

7. GELU (Gaussian Error Linear Unit)
torch.nn.GELU
ReLUとSigmoidの特性を組み合わせた新しい活性化関数。
数式: \( \text{GELU}(x) = 0.5x \left[ 1 + \tanh\left(\sqrt{\frac{2}{\pi}} (x + 0.044715x^3)\right)\right] \)
import torch
f=torch.nn.GELU()
out=f(torch.randn(4))
print(out)